from typing import *
import numpy as np
from qiskit.quantum_info import Statevector, Operator
from qiskit_aer import AerSimulator
from qiskit.visualization import plot_histogram
from qiskit import QuantumCircuit
sim = AerSimulator()
from util import zero, one
Foundations II: No Cloning#
The no cloning theorem is a claim that you cannot duplicate an arbitrary quantum state. In a multi-qubit system, this in some sense means that each qubit is unique. It is an important fact of quantum computation so we’ll give a proof.
References
Introduction to Classical and Quantum Computing: Chapter 4.4.4
Introduction to Quantum Information Science: Lecture 7 by Scott Aaronson
Cloning a single-qubit? Let’s try it …#
Suppose that we could construct a quantum circuit that clones a single qubit \(|\psi\rangle = \alpha |0\rangle + \beta |1\rangle\). Then we can find a unitary \(U\) such that
We want to show that it’s impossible to construct such a U.
Cloning is Impossible#
Unpack the input.
Unpack the output.
Thus
which is impossible since \(\alpha^2\) (similarly \(\alpha\beta\) and \(\beta^2\)) is not a linear combination of \(\alpha\) and \(\beta\) (when one of \(\alpha, \beta \neq 0\).
# We can also check the unitary condition
psi = np.array([1/np.sqrt(2)+0j, 0j, 1/np.sqrt(2)+0j, 0j])
U_psi = np.array([1/np.sqrt(2)+0j, 1/2+0j, 1/2+0j, 1/np.sqrt(2)+0j])
print("Does psi have unit norm:", np.allclose(1, np.linalg.norm(psi)))
print("Does U(psi) have unit norm:", np.allclose(1, np.linalg.norm(U_psi)))
Does psi have unit norm: True
Does U(psi) have unit norm: False
Doesn’t the CNOT gate copy?#
Recall that the CNOT gate acts as
Notably \(|00\rangle \mapsto |00\rangle\) and \(|10\rangle \mapsto |11\rangle\).
Why doesn’t this violate the no cloning theorem?
Indeed, the circuit below seems to succeed in copying \(1\) from \(|q_0\rangle\) to \(|q_1\rangle\).
qc_cnot = QuantumCircuit(2, 2)
qc_cnot.initialize(one, 0); qc_cnot.initialize(zero, 1)
qc_cnot.cx(0, 1) # 0 is control, 1 is target
qc_cnot.measure(0, 0)
qc_cnot.measure(1, 1)
qc_cnot.draw(output="mpl", style="iqp")
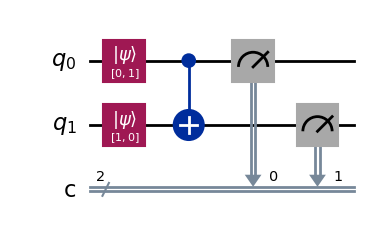
results = sim.run(qc_cnot, shots=1024).result()
plot_histogram(results.get_counts())
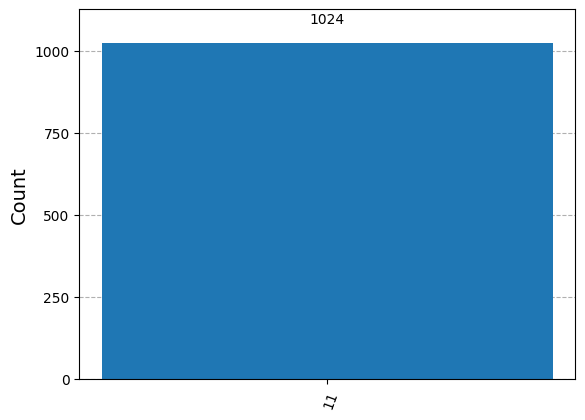
Answer#
The answer is that only classical information can be copied. In other words, We need to have a degenerate case where either \(\alpha=0\) or \(\beta=0\). Here’s an example where we cannot copy information.
qc_cnot = QuantumCircuit(2, 2)
qc_cnot.initialize(zero, 0)
# Trying to copy equal superposition
qc_cnot.initialize(1/np.sqrt(2)*(zero + one), 1)
qc_cnot.cx(0, 1) # 0 is control, 1 is target
qc_cnot.measure(0, 0)
qc_cnot.measure(1, 1)
qc_cnot.draw(output="mpl", style="iqp")
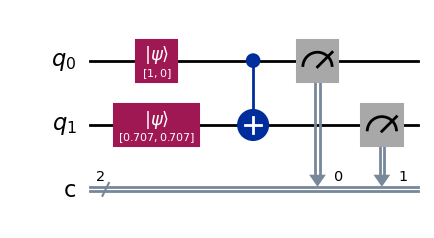
results = sim.run(qc_cnot, shots=1024).result()
answer = results.get_counts()
# Should expect 50% 00 and 50% 11 if we were able to copy successfully
plot_histogram(answer)
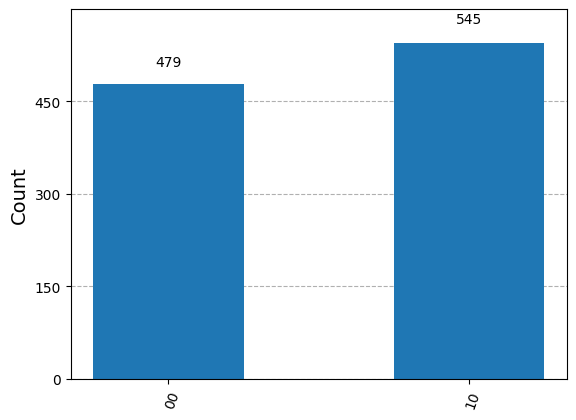
Optional: Another proof that cloning isn’t possible#
Recall that a unitary matrix \(U\) is one where
An equivalent characterization of a unitary matrix \(U\) is that it also preserves inner products, i.e.,
No-cloning via inner product#
This can only hold if \(\langle v, w \rangle = 0\) or \(\langle v, w \rangle = 1\), i.e., they are part of the same orthogonal basis.
Probabilistic version of no cloning#
The no cloning theorem is not unique to quantum computation. In particular, you also can’t clone an arbitrary probabilistic state if you don’t have access to the process that generated it.
Summary#
We stated and proved the no cloning theorem. It states that arbitrary quantum states cannot be copied.
Classical information can still be copied as demonstrated by the CNOT gate.