import numpy as np
import matplotlib.pyplot as plt
import time
from typing import *
from qiskit import QuantumCircuit
from qiskit_aer import AerSimulator
from qiskit.circuit.library.standard_gates import XGate
from qiskit.quantum_info import Statevector, Operator
from qiskit.visualization import plot_histogram, plot_bloch_multivector
sim = AerSimulator()
from util import zero, one
QI: Quantum Error Correction#
Throughout this series of notebooks, we have assumed logical qubits, i.e., qubits without errors. However, the qubits we have today cannot be controlled perfectly and thus have errors. These are called physical qubits. 2. We’ll first look at sources of error in a single qubit. 3. Then, we’ll look at how to use quantum error correction (QEC) to turn physical qubits into logical qubits. Specifically, we’ll look at the bit-flip code.
References
Sources of Error#
Recall a single qubit.
plot_bloch_multivector(zero)
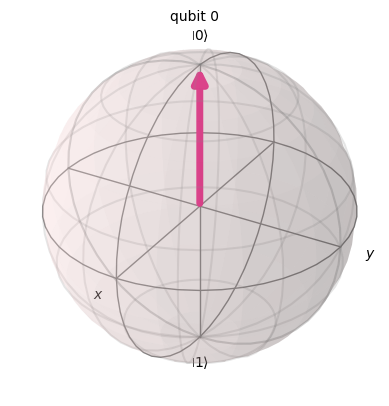
YZ-Error or Bit-Flip Error#
Instead of having a logical \(|0\rangle\), we may have a perturbed \(|0\rangle\) that is off in the \(YZ\) plane (rotation about x-axis) by a small angle.
In this example, consider throwing off the \(|0\rangle\) by a \(\pi/20\) angle.
qc_yz_err = QuantumCircuit(1)
qc_yz_err.rx(np.pi/20, 0)
qc_yz_err.draw(output="mpl", style="iqp")
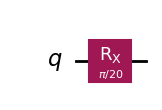
plot_bloch_multivector(zero.evolve(Operator(qc_yz_err)))
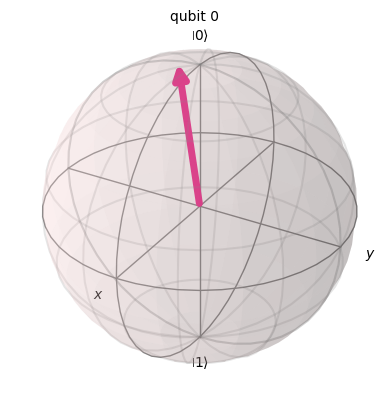
How bad is the error?#
We can compute the quantum state and compute the probability of measuring a \(|0\rangle\).
zero.evolve(Operator(qc_yz_err)).draw("latex")
# Probability of measuring a |0>
0.9969173337**2
0.9938441702315172
As a histogram#
qc = QuantumCircuit(1, 1)
qc.rx(np.pi/20, 0)
qc.measure(0, 0)
results = sim.run(qc, shots=1024).result()
answer = results.get_counts()
plot_histogram(answer)
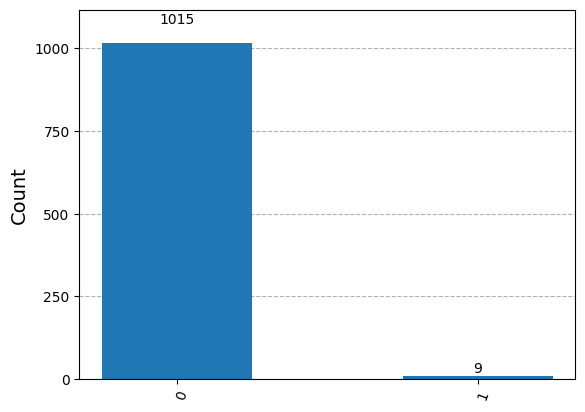
How small is small?#
You might think that \(0.9938441702315172\) is quite a high probability.
Let’s plot the number of successive operations we can perform and the probability of success without error.
For comparison, your classical computer can effectively run billions of operations without errors every second.
p = 0.9938441702315172
num_ops = range(1, 1000, 10)
prob_err = [p**x for x in num_ops]
plt.plot(num_ops, prob_err)
plt.title("Probability of success as a function of number of operations")
plt.ylabel("Probability");
plt.xlabel("Number of operations");
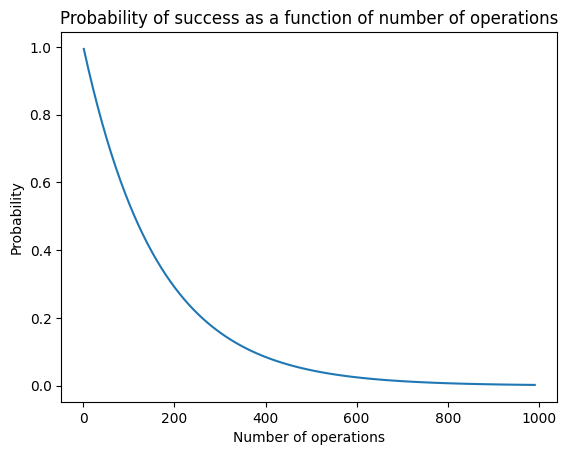
p = 0.999999 # Improving the error rate
num_ops = range(1, 1000, 10)
prob_err = [p**x for x in num_ops]
plt.plot(num_ops, prob_err)
plt.title("Probability of success as a function of number of operations")
plt.ylabel("Probability");
plt.xlabel("Number of operations");
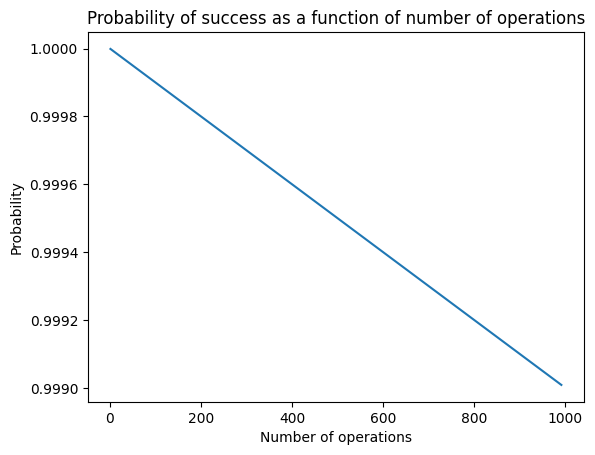
qc_yz_err2 = QuantumCircuit(1)
qc_yz_err2.rx(np.pi/1800, 0)
zero.evolve(Operator(qc_yz_err2)).draw("latex")
0.9999996192**2
0.999999238400145
# Your qubit needs to be accurate to within 0.1 degrees in order
# to perform 1000 operations with probability 0.999 of success
print("Angle in degrees", np.pi/1800*(180/np.pi))
Angle in degrees 0.1
XY-Error or Phase-Flip Error#
Unlike classical error correction where we can only flip a \(0\) to a \(1\), or vice versa, we also have phase-flip errors.
This is perturbation of a quantum state in the \(XY\)-plane, i.e., rotation about the Z-axis in the Bloch sphere.
Consider throwing off the \(H|0\rangle\) by a \(\pi/20\) angle in the \(XY\) plane. We are using \(H|0\rangle\) since rotation of \(|0\rangle\) in the z-axis.
qc_xy_err = QuantumCircuit(1)
qc_xy_err.h(0)
qc_xy_err.rz(np.pi/20, 0)
qc_xy_err.draw(output="mpl", style="iqp")
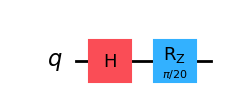
plot_bloch_multivector(zero.evolve(Operator(qc_xy_err)))
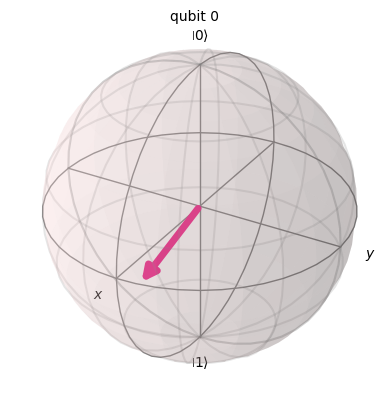
zero.evolve(Operator(qc_xy_err)).draw("latex")
# Probability of measuring a |0>
np.abs(0.704927007 - 0.0554789586*1j)**2
np.float64(0.5000000000453187)
Discusion question#
Is it possible to measure the error in this qubit? How would we be able to tell that there is an error in this qubit?
qc = QuantumCircuit(1, 1)
qc.h(0)
qc.rz(np.pi/20, 0)
qc.measure(0, 0)
results = sim.run(qc, shots=1024).result()
answer = results.get_counts()
plot_histogram(answer)
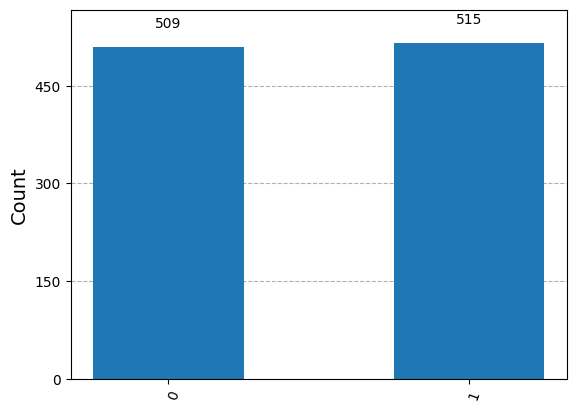
Bit-Flip Error and Phase-Flip Error#
A single qubit can exhibit both bit-flip and phase-flip errors.
qc_err = QuantumCircuit(1)
qc_err.rx(np.pi/20, 0)
qc_err.rz(np.pi/20, 0)
qc_err.draw(output="mpl", style="iqp")
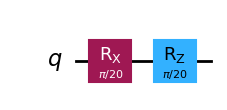
plot_bloch_multivector(zero.evolve(Operator(qc_err)))
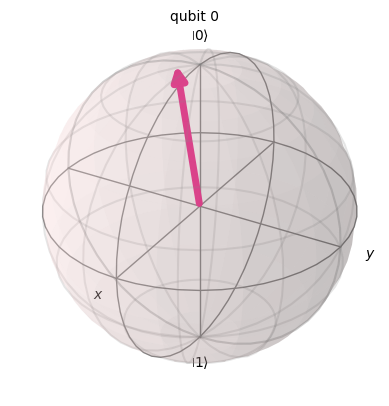
zero.evolve(Operator(qc_err)).draw("latex")
np.abs(0.9938441703 - 0.0782172325*1j)**2
np.float64(0.9938441702992548)
Histogram#
qc = QuantumCircuit(1, 1)
qc.rx(np.pi/20, 0)
qc.measure(0, 0)
results = sim.run(qc, shots=1024).result()
answer = results.get_counts()
plot_histogram(answer)
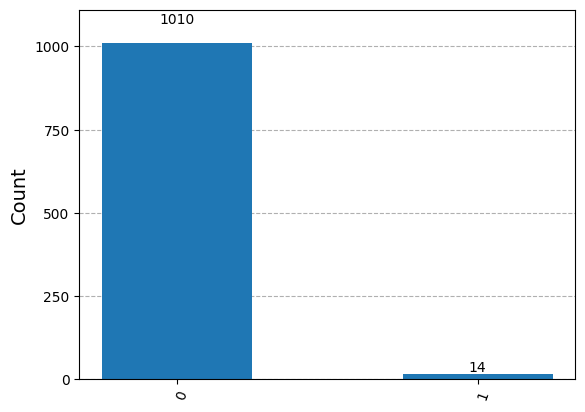
Error Correction Strategy#
Introduce Bit-Flip Code which corrects errors in the YZ plane of the Bloch sphere.
The Phase-Flip Code which corrects errors in the XY plane of the Bloch sphere is similar to the Bit-Flip code.
Shor’s Code combines the Bit-Flip Code and Phase-Flip Code to correct both flip and phase errors.
Bit-Flip Code#
In the Bit-Flip Code, we will use three physical qubits to encode one logical qubit.
Logical \(|0_L\rangle\) is defined as
Logical \(|1_L\rangle\) is defined as
Thus any logical qubit can be defined as
Example: Entire Qubit Flipped#
Suppose we have a qubit
where one of the underlying qubits becomes corrupted. For example,
so that the most-significant physical qubit becomes flipped.
Intuitively, our error-correction scheme is: predict the majority of a qubit in a group since flipping two \(|0\rangle\)’s to \(|1\rangle\)’s is less likely than flipping a single one.
However, how do we do this without measuring the qubit since doing so would destroy the state of superposition?
Majority Error-Correcting Code#
Suppose we have \(|b_2 b_1 b_0\rangle\).
We want \(|001\rangle\), \(|010\rangle\), and \(|100\rangle\) to be corrected to \(|000\rangle\).
Similarly we want \(|110\rangle\), \(|101\rangle\), and \(|011\rangle\) to be corrected to \(|111\rangle\).
Parity and Majority Error-Correcting Code#
We can accomplish this by computing the parity of adjacent bits \(b_2 \oplus b_1\) and \(b_1 \oplus b_0\).
Codeword |
b2 XOR b1 |
b1 XOR b0 |
---|---|---|
000 |
0 |
0 |
001 |
0 |
1 |
010 |
1 |
1 |
011 |
1 |
0 |
100 |
1 |
0 |
101 |
1 |
1 |
110 |
0 |
1 |
111 |
0 |
0 |
Parity of \(00\) means no error (either \(|000\rangle\) or \(|111\rangle\)).
Parity of \(01\) means \(b_0\) flipped (either \(|001\rangle\) or \(|110\rangle\)).
Parity of \(10\) means \(b_2\) flipped (either \(|011\rangle\) or \(|100\rangle\)).
Parity of \(11\) means \(b_1\) flipped (either \(|010\rangle\) or \(|101\rangle\)).
Crucially, we do not need to know the codeword! This means we can implement it in a quantum setting without measurement.
Quantum Parity and Majority Error-Correcting Code#
Recall the CNOT gate calculates an XOR.
We would thus like to construct a circuit \(U\) such that
qc_parity = QuantumCircuit(5)
qc_parity.cx(0, 3); qc_parity.cx(1, 3) # Compute |q_3> = |q_1 XOR q_0>
qc_parity.cx(1, 4); qc_parity.cx(2, 4) # Compute |q_4> = |q_2 XOR q_1>
qc_parity.draw(output="mpl", style="iqp")
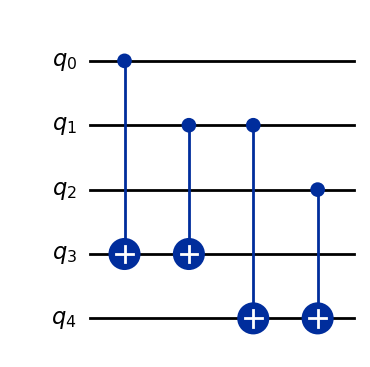
# |q_3 q_3 q_2 q_1 q_0>
# |q_2 q_1 q_0> = |000>
# |q_4 q_3> = |(q_2 XOR q_1) (q_1 XOR q_0)> = 00
# This means there are no errors
(zero ^ zero ^ zero ^ zero ^ zero).evolve(Operator(qc_parity)).draw("latex")
# |q_3 q_3 q_2 q_1 q_0>
# |q_2 q_1 q_0> = |010>
# |q_4 q_3> = |(q_2 XOR q_1) (q_1 XOR q_0)> = 11
# Parity of 11 means q_1 flipped (either |010> or |101>).
(zero ^ zero ^ zero ^ one ^ zero).evolve(Operator(qc_parity)).draw("latex")
# |q_3 q_3 q_2 q_1 q_0>
# |q_2 q_1 q_0> = |011>
# |q_4 q_3> = |(q_2 XOR q_1) (q_1 XOR q_0)> = 10
# Parity of 10 means q_2 flipped (either |011> or |100>).
(zero ^ zero ^ zero ^ one ^ one).evolve(Operator(qc_parity)).draw("latex")
Correcting the parity#
Recall that a parity of \(11\) means \(q_1\) flipped (either \(|010\rangle\) or \(|101\rangle\)). Thus we need a circuit
Recall that a parity of \(10\) means \(q_2\) flipped (either \(|011\rangle\) or \(|100\rangle\)). Thus we need a circuit
Finally recall that a parity of \(01\) means that \(q_0\) flipped (either \(|001\rangle\) or \(|110\rangle\)). Thus we need a circuit
qc_fix = QuantumCircuit(5)
qc_fix.append(XGate().control(2), [3, 4, 1]) # |11> -> flip q_1
qc_fix.barrier()
qc_fix.x(4); qc_fix.append(XGate().control(2), [3, 4, 0]); qc_fix.x(4) # |10> -> flip q_0
qc_fix.barrier()
qc_fix.x(3); qc_fix.append(XGate().control(2), [3, 4, 2]) # |01> -> flip q_2
qc_fix.draw(output="mpl", style="iqp")
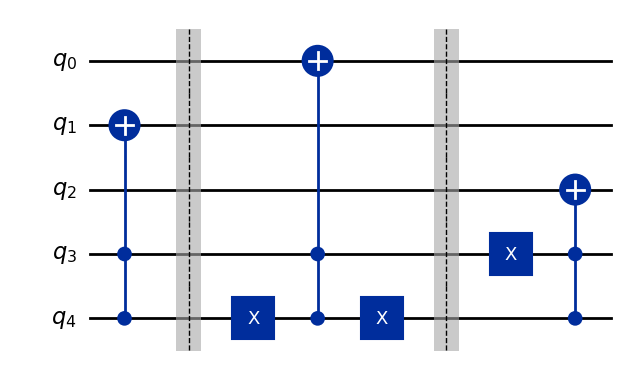
Putting it together#
def create(q_2, q_1, q_0):
qc = QuantumCircuit(5, 5)
qc.initialize(q_0, 0); qc.initialize(q_1, 1); qc.initialize(q_2, 2);
qc.barrier()
# Compute parity
qc.cx(0, 3); qc.cx(1, 3) # Compute |q_3> = |q_1 XOR q_0>
qc.cx(1, 4); qc.cx(2, 4) # Compute |q_4> = |q_2 XOR q_1>
qc.barrier()
qc.measure(3, 3); qc.measure(4, 4)
qc.barrier()
# Fix parity
qc.append(XGate().control(2), [3, 4, 1]) # |11> -> flip q_1
qc.barrier()
qc.x(4); qc.append(XGate().control(2), [3, 4, 0]); qc.x(4) # |10> -> flip q_0
qc.barrier()
qc.x(3); qc.append(XGate().control(2), [3, 4, 2]) # |01> -> flip q_2
qc.barrier()
# Reinitialize for further error correction
qc.initialize(zero, 3)
qc.initialize(zero, 4)
# Readout
qc.measure(0, 0); qc.measure(1, 1); qc.measure(2, 2) # Measure result
return qc
qc = create(one, one, zero)
qc.draw(output="mpl", style="iqp")
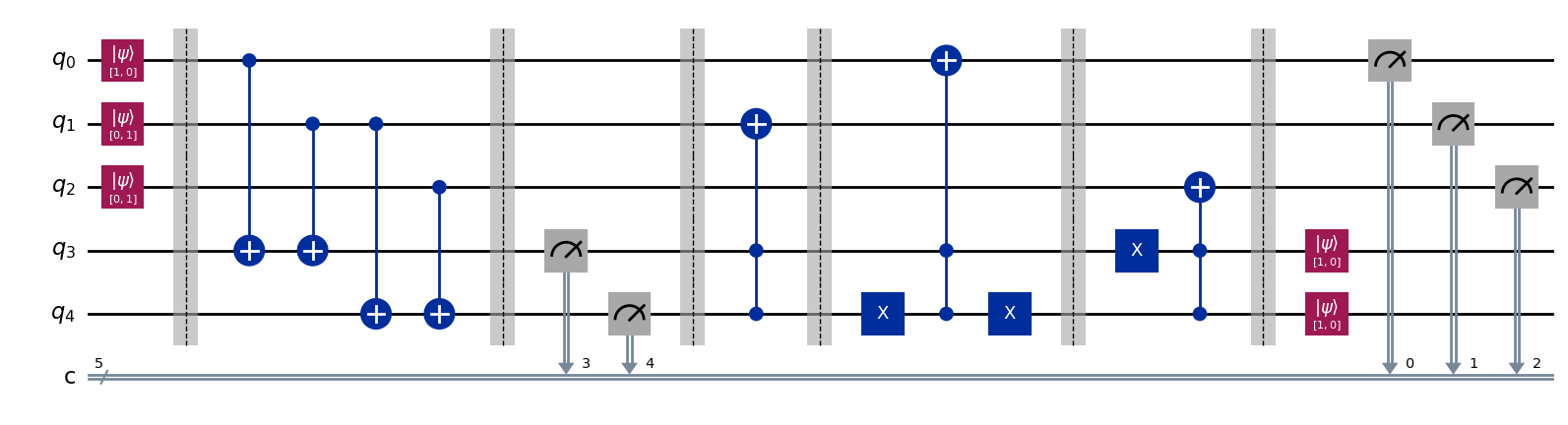
results = sim.run(qc, shots=1024).result()
answer = results.get_counts()
# Note that |01111> = |01> \oplus |111> so that |110> got corrected to |111>
plot_histogram(answer)
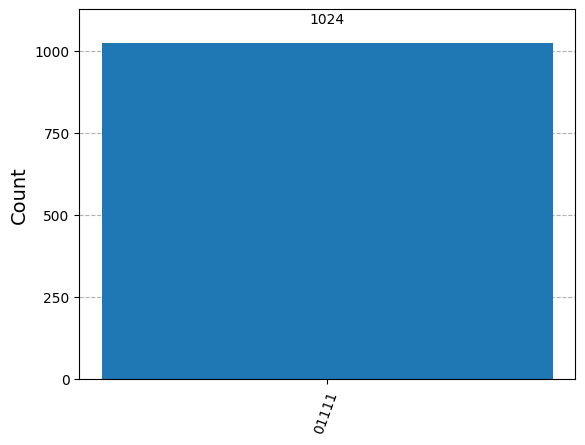
qc = create(zero, one, zero)
results = sim.run(qc, shots=1024).result()
answer = results.get_counts()
# Note that |11000> = |11> \oplus |000> so that |010> got corrected to |000>
plot_histogram(answer)
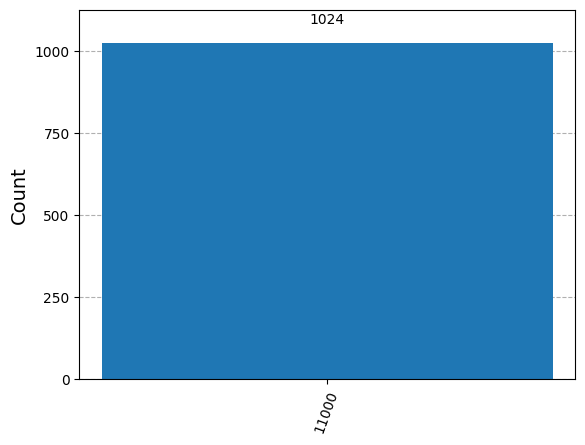
What about partial errors?#
Suppose we corrupt a qubit by a rotation of \(\theta\) about the x-axis (\(YZ\) plane). This corresponds to using the \(R_x(\theta)\) gate.
Reparameterizing as \(\epsilon = \sin (\theta/2)\), we obtain
Example: suppose most-significant qubit is corrupted#
This produces a physical qubit in state
\(parity(q_2, q_1) = 0\) and \(parity(q_1, q_0) = 0\) occurs with the state
This occurs with probability \(|\alpha\sqrt{1 - \epsilon^2}|^2 + |\beta\sqrt{1 - \epsilon^2}|^2 = 1 - \epsilon^2\). Thus, with probability \(1 - \epsilon^2\), we do not need to do anything.
\(parity(q_2, q_1) = 1\) and \(parity(q_1, q_0) = 0\) occurs with the state
This occurs with probability \(|-i\beta\epsilon|^2 + |-i\alpha\epsilon|^2 = \epsilon^2\). Thus, with probability \(\epsilon^2\), we should apply an \(X\) gate to the most-significant qubit.
The reasoning for partial flips on the middle and least significant qubit is similar.
Thus this procedure is exactly the same as the complete flip case.
def corrupt(angle, q):
qc_err = QuantumCircuit(1)
# qc_err.p(np.pi, 0)
qc_err.rx(angle * np.pi/180, 0)
return q.evolve(Operator(qc_err))
plot_bloch_multivector(corrupt(90, zero))
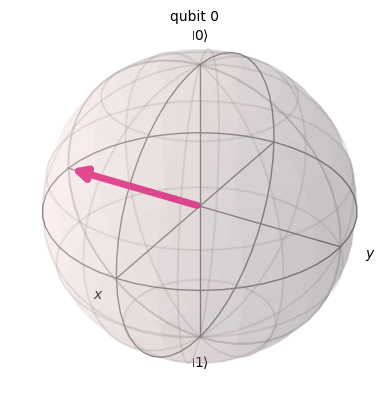
qc = create(one, one, corrupt(90, one))
results = sim.run(qc, shots=1024).result()
answer = results.get_counts()
plot_histogram(answer)
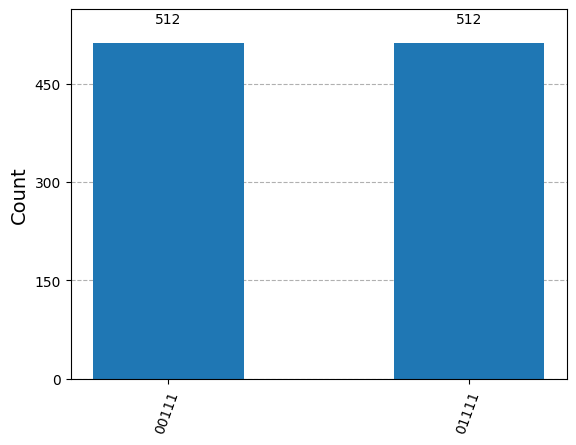
Summary#
We saw the basics of quantum error correction based on bit-flip code.
The bit-flip code encodes 1 logical qubit with 3 physical qubits. 2 ancila (additional) qubits in addition to mid-circuit measurement were needed to complete the code.
Correcting bit-flips and phase-flips together can be done with Shor’s code (see Introduction to Classical and Quantum Computing, Chapter 4.7 for more details)
It is an active area of research of how to develop better quantum error correcting codes that have lower overhead or can correct different kinds of errors.
Logical qubits are required to implement Grover’s search and Shor’s algorithm